PHP 1.26 - How To Work With Arrays In PHP
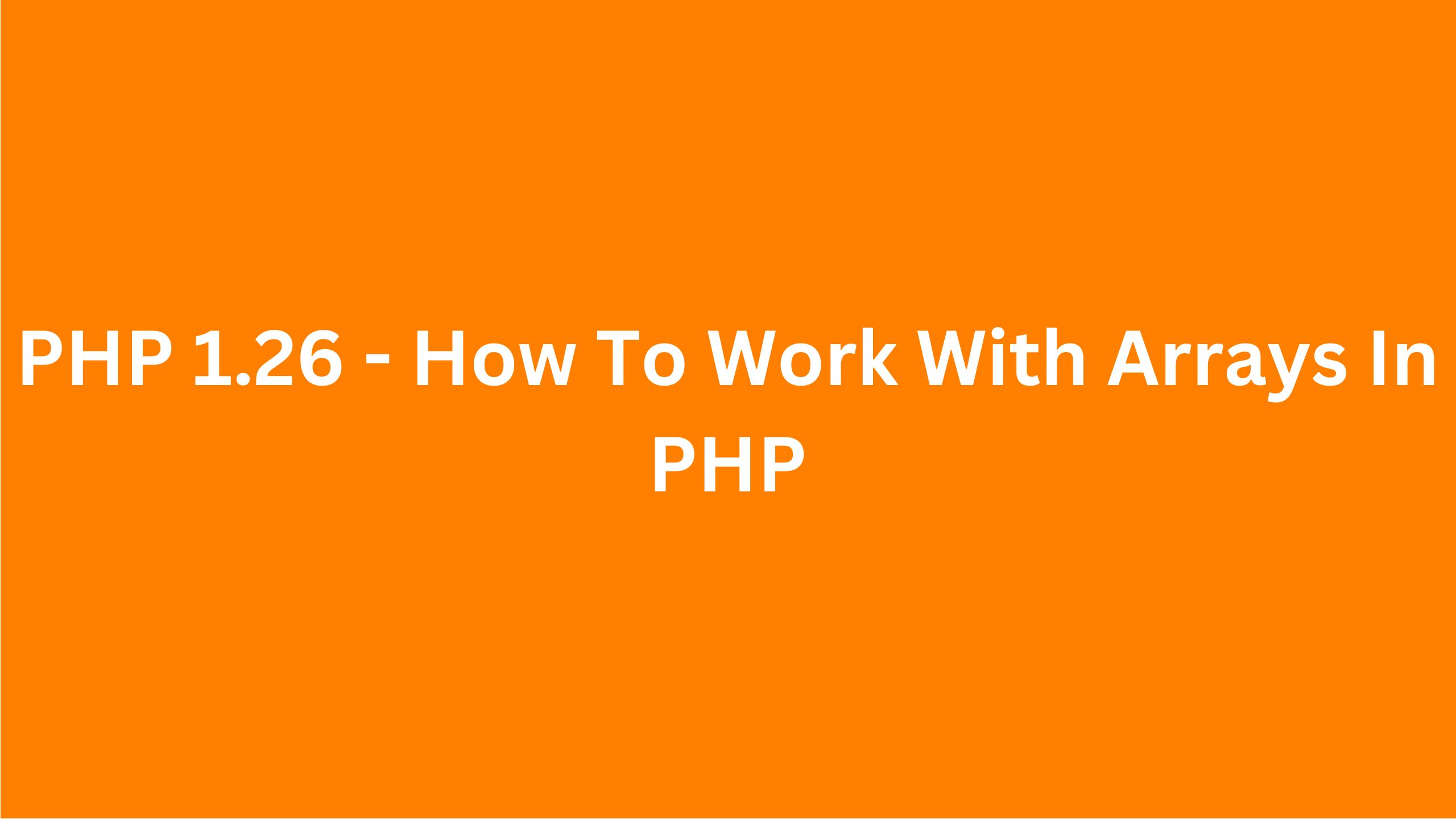
PHP has a lot of built-in array functions to work with arrays, and you have already seen me use some of these array functions in previous lessons. But in this lesson, let's go over some of the other important functions that I think will come in handy later, and you will see me use from time to time. I will also leave the link to page in the description where you can check the rest of the built-in array functions. As you can see, there are a lot of them.
First, we have array_chunk()
. Sometimes you might want to split an array into chunks of arrays with a specified length. The first argument that array_chunk()
accepts is the array, the second argument is the length, and then the third argument is optional, which is whether you want to preserve keys or not. So let's see what happens:
<?php
require 'helpers.php';
$items = ['a' => 1, 'b' => 2, 'c' => 3, 'd' => 4, 'e' => 5];
echo '<pre>';
print_r(array_chunk($items, 2));
Array
(
[0] => Array
(
[0] => 1
[1] => 2
)
[1] => Array
(
[0] => 3
[1] => 4
)
[2] => Array
(
[0] => 5
)
)
So if we refresh the page, we see the array of size three, and each of that array contains two elements from the original array.
Now, as you notice, the keys were not preserved, right? Because the keys are zero, one, instead of 'a' and 'b'. If you want to preserve the keys, you need to pass in the third argument as true:
<?php
$items = ['a' => 1, 'b' => 2, 'c' => 3, 'd' => 4, 'e' => 5];
echo '<pre>';
print_r(array_chunk($items, 2, true));
Array
(
[0] => Array
(
[a] => 1
[b] => 2
)
[1] => Array
(
[c] => 3
[d] => 4
)
[2] => Array
(
[e] => 5
)
)
Next, we have array_combine()
. This function creates an array from the given keys and values. It will throw an error if the number of elements in the first array doesn't match the number of elements in the second array. So basically, it will use the values from the array 1 as the keys, and it will use the values from the array 2 as the values, and it will create the new array:
<?php
$array1 = ['a', 'b', 'c'];
$array2 = [5, 10, 15];
echo '<pre>';
print_r(array_combine($array1, $array2));
Array
(
[a] => 5
[b] => 10
[c] => 15
)
If we refresh the page, here we are getting an array where the keys are a, b, and c, and values are 5, 10, and 15. If size did not match, you would throw an error. Note that prior to PHP 8, this would throw just a warning and return false instead. But in PHP 8, most internal functions now throw an error instead of the warning when parameter validation fails.
Next, we have array_filter()
, which iterates over each array value and it passes the value to the given callback. If the return of that callback is true, then the value is returned into the resulting array. Otherwise, the element will be discarded. For example, let's say that we wanted to filter out the odd numbers from the array and only keep the even numbers:
<?php
$array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
$even = array_filter($array, fn($number) => $number % 2 === 0);
echo '<pre>';
print_r($even);
Array
(
[1] => 2
[3] => 4
[5] => 6
[7] => 8
[9] => 10
)
As mentioned before, by default, the value is passed to the callback as the argument, but you could change that by specifying the mode flag as the third parameter. So you could specify "ARRAY_FILTER_USE_KEY", and now instead of "number" being passed as the value, it will be passed as the key:
<?php
$array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
$even = array_filter($array, fn($number) => $number % 2 === 0, ARRAY_FILTER_USE_KEY);
echo '<pre>';
print_r($even);
Array
(
[0] => 1
[2] => 3
[4] => 5
[6] => 7
[8] => 9
)
Or you could use "ARRAY_FILTER_USE_BOTH", and now the first argument is the value and the second argument is the key:
<?php
$array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
$even = array_filter($array, fn($number) => $number % 2 === 0, ARRAY_FILTER_USE_BOTH);
echo '<pre>';
print_r($even);
Array
(
[1] => 2
[3] => 4
[5] => 6
[7] => 8
[9] => 10
)
Notice how the keys of these elements have gaps in between them. That's because array keys are not preserved, so it might result in gaps like this if the array is numerically indexed. If you want to re-index the array after filtering it, you need to use a function called array_values()
, which will simply return all values of the array indexed numerically:
<?php
$array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
$even = array_filter($array, fn($number) => $number % 2 === 0, ARRAY_FILTER_USE_BOTH);
$even = array_values($even);
echo '<pre>';
print_r($even);
Array
(
[0] => 2
[1] => 4
[2] => 6
[3] => 8
[4] => 10
)
Now, if we print it, it's indexed correctly.
Similar to array_values()
, which returns the values of the arrays, we could use array_keys()
to get the keys of the array. You could also search for specific values and only return the keys for the values that match. For example:
<?php
$array = ['a' => 5, 'b' => 10, 'c' => 15, 'd' => 5, 'e' => 10];
$keys = array_keys($array);
echo '<pre>';
print_r($keys);
Array
(
[0] => a
[1] => b
[2] => c
[3] => d
[4] => e
)
We could specify the search value and say that we want to search for 10, it's going to return 'b' and 'e' because that's where the value 10 is found:
<?php
$array = ['a' => 5, 'b' => 10, 'c' => 15, 'd' => 5, 'e' => 10];
$keys = array_keys($array, '10');
echo '<pre>';
print_r($keys);
Array
(
[0] => b
[1] => e
)
Note that it does the loose comparison by default, but it could do the strict comparison by passing "true" as the third argument.
array_map()
is one of the other functions that you will probably use more often. Like array_filter()
, it simply applies the callback to each element of the given array. For example, let's say we had an array of numbers and we want to multiply each of these values by three. We could do that by using array_map()
and pass the callback:
<?php
$array = [1, 2, 3, 4, 5, 6];
$array = array_map(fn($number) => $number *= 3, $array);
echo '<pre>';
print_r($array);
Array
(
[0] => 3
[1] => 6
[2] => 9
[3] => 12
[4] => 15
[5] => 18
)
Now, let me show you an example with multiple arrays where we also have custom keys:
<?php
$array1 = ['a' => 1, 'b' => 2, 'c' => 3];
$array2 =['d' => 4, 'e' => 5, 'f' => 6];
$array = array_map(fn($number1, $number2) => $number1 * $number2, $array1, $array2);
echo '<pre>';
print_r($array);
Array
(
[0] => 4
[1] => 10
[2] => 18
)
But note that now the array has been re-indexed numerically, and it no longer has the string keys. That's because we're passing more than one array. Note that if you're passing only a single array to the array_map()
function, it will preserve the keys. However, if you are passing more than one array, then it will re-index the array numerically. Also, note that when doing array_map()
on multiple arrays, it is best to have arrays of the same length because the callback will be applied in parallel. If you have uneven lengths, then the shorter array will be extended with empty elements. You could also pass null as the callback, and what that will do is that it will simply build up an array from the given arrays.
Next, we have array_merge()
that allows you to merge multiple arrays. If arrays have the same numeric keys, then it will not overwrite the values, but instead, they will be appended. Also, numeric keys will be re-indexed starting from zero:
<?php
$array1 = [1, 2, 3];
$array2 = [4, 5, 6];
$array3 = [7, 8, 9];
$merged = array_merge($array1, $array2, $array3);
echo '<pre>';
print_r($merged);
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
[4] => 5
[5] => 6
[6] => 7
[7] => 8
[8] => 9
)
For example, in this case, I have three arrays where I don't specify the keys, it's just the values. And when I merge and I refresh, it will simply append at the end, and we're going to get all items from all the arrays.
However, if you have the same string keys, then the later value will overwrite the previous one. For example:
<?php
$array1 = [1, 2, 3];
$array2 = ['a' => 4, 'b' => 5, 'c' => 6];
$array3 = [7, 8, 9, 'b' => 10];
$merged = array_merge($array1, $array2, $array3);
echo '<pre>';
print_r($merged);
Array
(
[0] => 1
[1] => 2
[2] => 3
[a] => 4
[b] => 10
[c] => 6
[3] => 7
[4] => 8
[5] => 9
)
Another really useful function is array_reduce()
, which reduces the array to a single value using the callback function that you passed. For example, let's say we have an array, and we wanted to run some calculations where we multiply the price by quantity and sum it all up to get the total invoice price. Now, of course, you could do that many other ways, but one way is to use array_reduce()
:
<?php
$invoiceItems = [
['price' => 9.99, 'qty' => 3, 'desc' => 'Item 1'],
['price' => 29.99, 'qty' => 1, 'desc' => 'Item 2'],
['price' => 149, 'qty' => 1, 'desc' => 'Item 3'],
['price' => 14.99, 'qty' => 2, 'desc' => 'Item 4'],
['price' => 4.99, 'qty' => 4, 'desc' => 'Item 5'],
];
$total = array_reduce($invoiceItems, fn($sum, $item) => $sum + $item['qty'] * $item['price']);
echo $total;
258.9
You could also start off the initial value as a third parameter with something like 500. So if you refresh, it's going to be 758.9:
<?php
$invoiceItems = [
['price' => 9.99, 'qty' => 3, 'desc' => 'Item 1'],
['price' => 29.99, 'qty' => 1, 'desc' => 'Item 2'],
['price' => 149, 'qty' => 1, 'desc' => 'Item 3'],
['price' => 14.99, 'qty' => 2, 'desc' => 'Item 4'],
['price' => 4.99, 'qty' => 4, 'desc' => 'Item 5'],
];
$total = array_reduce($invoiceItems, fn($sum, $item) => $sum + $item['qty'] * $item['price'], 500);
echo $total;
758.9
You could search the array by using array_search()
, which will return the key of the first matching value. So let's create an array as an example, and let's say we want to find the key of the letter "b.":
<?php
$array = ['a', 'b', 'c', 'D', 'E', 'ab', 'bc', 'cd', 'b', 'd'];
$key = array_search('b', $array);
echo $key;
1
Note that the letter "b" also appears second time at the end of the array, but it only returns the key of the first matching value, and in this case, that's 1. The search is also case-sensitive, so searching for uppercase "d" will print 3 and not 9, for example. array_search()
will return false if it cannot find the value, but it can return zero if it finds the matching value at the zeroth position. So you should be careful when you're doing comparisons. You should always use the strict comparison.
Alternatively, you could use another way to check if an item exists in the array, which is in_array()
to check if the value exists in an array. And instead of returning the key, it will just return a boolean, true or false:
<?php
$array = ['a', 'b', 'c', 'D', 'E', 'ab', 'bc', 'cd', 'b', 'd'];
$key = array_search('a', $array);
if (in_array('a', $array)) {
echo 'Letter a is in array';
}
Letter a is in array
Another function to find the difference between arrays by comparing values is array_diff()
. Sometimes you might want to find the difference between the arrays. There are quite a few functions that can help you do that. For example, one of them is array_diff()
and what it will do is that it will compare the first array against the rest of the given arrays and will return the values from the first array that are not present in any of the other arrays:
<?php
$array1 = ['a' => 1, 'b' => 2, 'c' => 3, 'd' => 4, 'e' => 5];
$array2 = ['f' => 4, 'g' => 5, 'i' => 6, 'j' => 7, 'k' => 8];
$array3 = ['l' => 3, 'm' => 9, 'n' => 10];
echo '<pre>';
print_r(array_diff($array1, $array2, $array3));
Array
(
[a] => 1
[b] => 2
)
We get 1 and 2. So 4 and 5 from the first array also appear in the second array, and the number 3 appears in the third array. So, therefore, 3, 4, and 5 are discarded because they're found in the other arrays, and only 1 and 2 are kept, and that's what we see on the screen.
If you want to find the difference between arrays by comparing both keys and values, you can use array_diff_assoc()
, array_diff()
only checks the values and not the keys. If you want to also check for the keys, you will need to use another function called array_diff_assoc()
:
<?php
$array1 = ['a' => 1, 'b' => 2, 'c' => 3, 'd' => 4, 'e' => 5];
$array2 = ['f' => 4, 'g' => 5, 'i' => 6, 'j' => 7, 'k' => 8];
$array3 = ['l' => 3, 'm' => 9, 'n' => 10];
echo '<pre>';
print_r(array_diff_assoc($array1, $array2, $array3));
Array
(
[a] => 1
[b] => 2
[c] => 3
[d] => 4
[e] => 5
)
If we uncomment that and refresh the page, now we're getting "a," "b," "c," "d," and "e." So right now, we're getting all of the elements from the array because none of these appear in the other arrays as the key-value pair.
You could also use array_diff_key()
to compute the difference only on keys instead of the values.
There are also plenty of functions available to help you sort arrays. Here are some of them - we have a function called asort()
which will sort the array by values. And you can pass a different sorting flag if needed:
<?php
$array = ['d' => 3, 'b' => 1, 'c' => 4, 'a' => 2];
asort($array);
echo '<pre>';
print_r($array);
Array
(
[b] => 1
[a] => 2
[d] => 3
[c] => 4
)
If you wanted to sort by keys, we have a function called ksort()
and this will now sort by keys instead of the values. And if we refresh, now it's sorted by keys:
<?php
$array = ['d' => 3, 'b' => 1, 'c' => 4, 'a' => 2];
ksort($array);
echo '<pre>';
print_r($array);
Array
(
[a] => 2
[b] => 1
[c] => 4
[d] => 3
)
Note that some of these array functions don't return a new array. Instead, they return a boolean and they take the array argument as reference, so it modifies the original variable.
Another useful sorting function is usort()
where you can pass a custom callback and sort it whichever way you want. So for example, we could do usort()
, and as the second argument, it accepts a callback. So we can do "fn" and it gets two values as the arguments, and we'll call them $a
and $b
We need to return zero if $a
and $b
are equal, we need to return a number less than zero or minus one when $a
is less than $b
and we need to return a number greater than zero or 1 when $a
is greater than $b.
Now, you can do this manually, of course, but there is a spaceship operator which we covered before, and it's a perfect use case for this. So we can do $a <=> $b
:
<?php
$array = ['d' => 3, 'b' => 1, 'c' => 4, 'a' => 2];
usort($array, fn($a, $b) => $a <=> $b);
echo '<pre>';
print_r($array);
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
)
And now, if we refresh the page, it's going to sort it numerically. We could also replace with $b <=> $a
and it's going to sort in reverse order:
<?php
$array = ['d' => 3, 'b' => 1, 'c' => 4, 'a' => 2];
usort($array, fn($a, $b) => $b <=> $a);
echo '<pre>';
print_r($array);
Array
(
[0] => 4
[1] => 3
[2] => 2
[3] => 1
)
Finally, let's move on to array destructuring. You can pull variables out of an array or destructure the array into separate variables. For example, let's say that we have this array of numbers [1, 2, 3, 4]
. We could destructure this and assign them to variables in one line. We could use a language construct called "list" or a shorthand version of it, which is just the square brackets like that:
<?php
$array = [1, 2, 3, 4];
[$a, $b, $c, $d] = $array;
echo $a . ', ' . $b . ', ' . $c . ', ' . $d;
1, 2, 3, 4
Now, even though "list" is not a built-in function and instead it's a language construct, I still wanted to cover this in this lesson. So, as you notice, it destructures the array into separate variables.
You can also skip elements by simply removing them and keeping the comma:
<?php
$array = [1, 2, 3, 4];
[$a, , $c, $d] = $array;
echo $a . ', ' . $c . ', ' . $d;
1, 3, 4
You can also destructure nested arrays. For example:
<?php
$array = [1, 2, [3, 4]];
[$a, $b, [$c, $d]] = $array;
echo $a . ', ' . $b . ', ' . $c . ', ' . $d;
1, 2, 3, 4
You can also specify keys:
<?php
$array = [1, 2, 3];
[1 => $a, 0 => $b, 2 => $c] = $array;
echo $a . ', ' . $b . ', ' . $c;
2, 1, 3
There are more array functions, but I cannot cover them all. I encourage you to check the link at the end of the lesson, if you want to know more.
Array Functions https://www.php.net/manual/en/ref.array.php