PHP 1.25 - How To Work With Dates & Time Zones
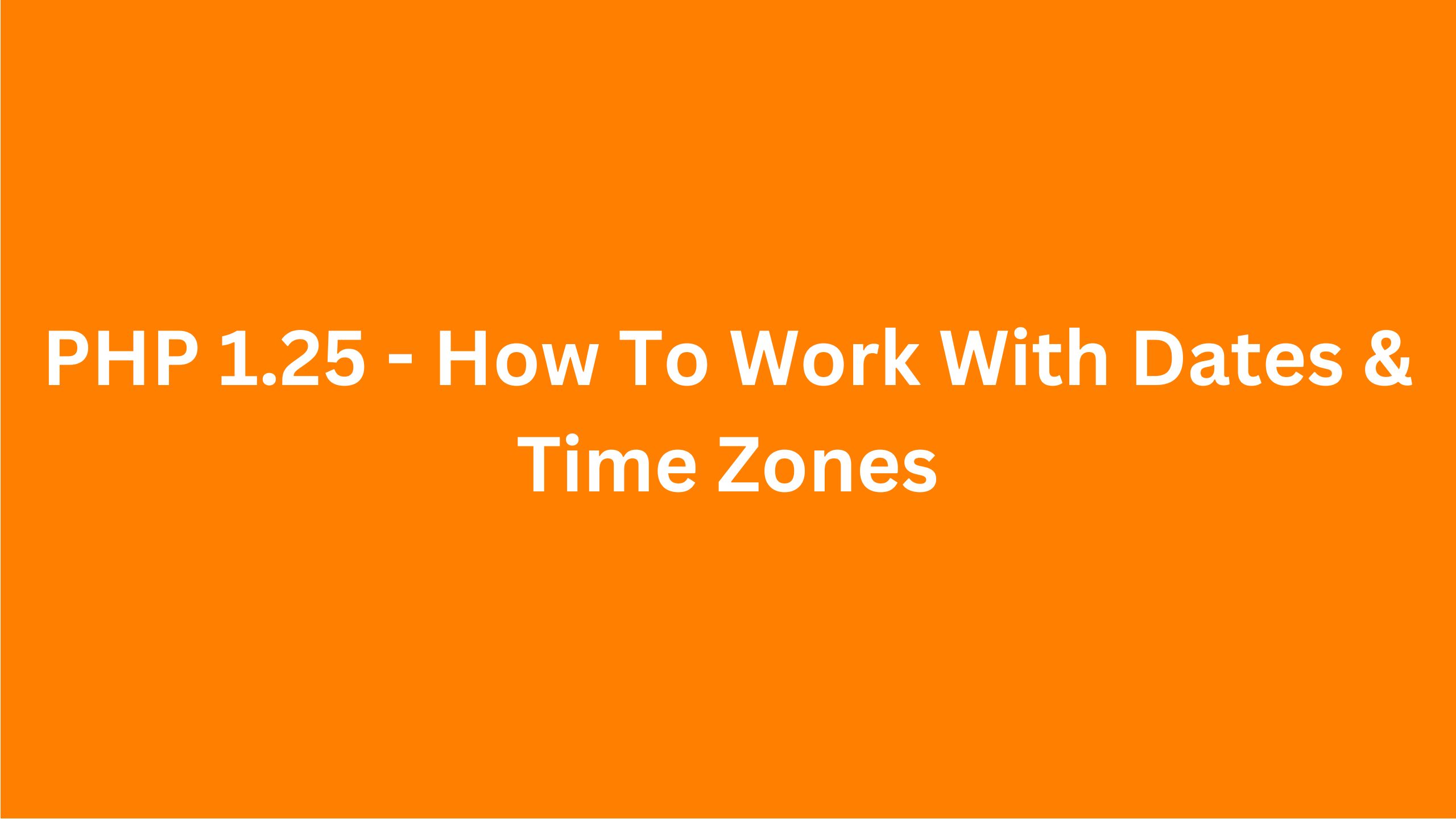
You will eventually need to work with date, time, and time zones in PHP. And as painful as it is to work with dates and times and time zones, it is an important topic. In this lesson, we'll cover the basic usage of date and time, and in the second section, we'll cover the object-oriented version using the DateTime class.
So first, we have the time()
function. So if we do:
<?php
echo time();
1683746077
This prints a large integer, and this integer is a Unix timestamp in seconds. So it's the number of seconds since 1970, January 1st. You could add the number of seconds to this timestamp to give you the time in the future or subtract the number of seconds to get the time in the past.
So for example, we could say:
<?php
$current_time = time();
echo $current_time . "<br>";
echo $current_time + 5 * 24 * 60 * 60 . "<br>";
1683746346
1684178346
So what we are essentially doing here is that this calculation here is converting 5 days into the number of seconds. So we're multiplying 5 by 24 hours, then multiplying the number of hours by 60 to convert it into the number of minutes and multiplying the total number of minutes by 60 to convert it into the number of seconds. And then we just simply add that value to the current time. And if we refresh, we see that the first one is the current time and the second is the time in five days.
And we could also subtract times. So we could subtract one day to get yesterday's time:
<?php
$current_time = time();
echo $current_time . "<br>";
echo $current_time + 5 * 24 * 60 * 60 . "<br>";
echo $current_time - 60 * 60 * 24 . "<br>";
So if we refresh, we have the current timestamp, we have the timestamp five days in the future, and we have the timestamp of yesterday.
Now, as useful as this is, we can actually format this into a date because you cannot just display the number of seconds to the user. Right? We can use a formatting dates function called date()
, which accepts the date format and optional timestamp as arguments. As the first argument, we need to pass in the format, and the second argument is optional, and we can pass in the timestamp. If we don't pass in anything for the second argument, it will just use the current time. For a list of supported formats, we could refer to the PHP documentation, which I'll share a link at the and of the lesson. So it's the PHP docs for the date time format, and here we see the available format characters. So the lowercase d
gives us the day of the month with the leading zero, so it's two digits. The uppercase D
gives us a textual representation of the day, and so on. So let's pick a few of these and test it out. So I'm going to do month/day here and then hour:minute and am/pm indicator:
<?php
$current_time = time();
echo $current_time . "<br>";
echo $current_time + 5 * 24 * 60 * 60 . "<br>";
echo $current_time - 60 * 60 * 24 . "<br>";
echo date('m/d/Y g:ia') . '<br>';
1683746781
1684178781
1683660381
05/10/2023 10:26pm
Now, as mentioned, we could pass in the timestamp as the second argument:
<?php
$current_time = time();
echo $current_time . "<br>";
echo $current_time + 5 * 24 * 60 * 60 . "<br>";
echo $current_time - 60 * 60 * 24 . "<br>";
echo date('m/d/Y g:ia') . '<br>';
echo date('m/d/Y g:ia', $current_time + 5 * 24 * 60 * 60) . '<br>';
1683746847
1684178847
1683660447
05/10/2023 10:27pm
05/15/2023 10:27pm
As you see, this is five days in the future.
Working with time zones, by default, all date and time functions use the time zone that is set in PHP's configuration file, but you could override that by using one of PHP's built-in functions at runtime. Right now, times are in my local time zone, but if I wanted to convert that to a different time zone, I could do date_default_timezone_set()
and we can pass it a valid time zone. You could look up the supported valid time zones in the PHP documentation. I will leave the link after the lesson.
So let's set it to UTC:
<?php
date_default_timezone_set('UTC');
$current_time = time();
echo $current_time . "<br>";
echo $current_time + 5 * 24 * 60 * 60 . "<br>";
echo $current_time - 60 * 60 * 24 . "<br>";
echo date('m/d/Y g:ia') . '<br>';
echo date('m/d/Y g:ia', $current_time + 5 * 24 * 60 * 60) . '<br>';
1683748427
1684180427
1683662027
05/10/2023 7:53pm
05/15/2023 7:53pm
We can also echo out the current time zone with date_default_timezone_get()
:
<?php
date_default_timezone_set('UTC');
$current_time = time();
echo $current_time . "<br>";
echo $current_time + 5 * 24 * 60 * 60 . "<br>";
echo $current_time - 60 * 60 * 24 . "<br>";
echo date('m/d/Y g:ia') . '<br>';
echo date('m/d/Y g:ia', $current_time + 5 * 24 * 60 * 60) . '<br>';
echo date_default_timezone_get();
1683748499
1684180499
1683662099
05/10/2023 7:54pm
05/15/2023 7:54pm
UTC
It is up to you what default time zone you want to set in your PHP configuration file, but I would highly recommend setting it to UTC. That way, it makes it easy for you to manage multiple time zones, and you could do the conversions whenever you're displaying the time to the user.
You can use the mktime()
function to get the Unix timestamp value. Use a function called mktime()
to get the Unix timestamp based on the arguments that you pass like hour, year, month, and so on. So we could say:
<?php
$mktimeTimestamp = mktime(0, 0, 0, 4, 10, null);
echo date('m/d/Y g:ia', $mktimeTimestamp) . "<br>";
04/10/2023 12:00am
We refresh, we get the fourth month, 10th day, current year.
You could also convert a string parsing dates using function strtotime()
. Convert the representation of a date into a Unix timestamp using the strtotime()
function. So, for example:
<?php
echo strtotime('2021-01-18 07:00:00');
1610946000
We could also format this to whatever format we want.
Now, in addition to parsing dates like this, you could also parse some relative formats like "tomorrow," for example:
<?php
echo date('m/d/Y g:ia', strtotime('tomorrow'));
05/11/2023 12:00am
And we refresh, we get tomorrow at midnight.
We could also do something like "first day of February.":
<?php
echo date('m/d/Y g:ia', strtotime('first day of february'));
02/01/2023 12:00am
You could parse dates using function date_parse()
and date_parse_from_format()
:
<?php
$date = date('m/d/Y g:ia', strtotime('first day of february'));
echo '<pre>';
var_dump(date_parse($date));
array(12) {
["year"]=>
int(2023)
["month"]=>
int(2)
["day"]=>
int(1)
["hour"]=>
int(0)
["minute"]=>
int(0)
["second"]=>
int(0)
["fraction"]=>
float(0)
["warning_count"]=>
int(0)
["warnings"]=>
array(0) {
}
["error_count"]=>
int(0)
["errors"]=>
array(0) {
}
["is_localtime"]=>
bool(false)
}
As you can see, we're getting a lot more details about that date. You could also use date_parse_from_format(), which will parse the date from a specific format. So you need to give in the format:
<?php
$date = date('m/d/Y g:ia', strtotime('first day of february'));
echo '<pre>';
var_dump(date_parse_from_format('m/d/Y g:ia', $date));
When we refresh, we get the same data, but if we were to give it the wrong format, we will get the wrong result with some errors populated in the array.
Date Formats - https://www.php.net/manual/en/datetime.format.php
Time Zones - https://www.php.net/manual/en/timezones.php
Relative Formats - https://www.php.net/manual/en/timezones.php